In case you missed it, make sure to read Announcing .NET 2015 – .NET as Open Source, .NET on Mac and Linux, and Visual Studio Community because there’s been some big stuff going on.
Here’s the summary of the .NET 2015 Wave of awesomeness.
- We are running .NET as an open source project going forward. http://github.com/dotnet
- There’s a home for Microsoft on GitHub now at http://microsoft.github.io
- You can get Visual Studio Community for FREE now at http://visualstudio.com/free
- ASP.NET 5 will run on Windows, Linux, and Mac. Yes, really.
The other thing I wanted to talk about is a newly organized group of technologies called OmniSharp. Just to be sure there’s no confusion, OmniSharp isn’t a Microsoft project. While there are two Microsoft folks on the team of 8 or so, we are working on it as community members, not in an official capacity.
I “launched” this project in my talk at the Connect() mini-conference in New York a few weeks back. You can watch that video here on Channel 9 now if you like. However, the technologies around and under OmniSharp have been around for years…like over a decade!
As a team and a community we pulled together a bunch of projects and plugins, got organized, and created https://github.com/omnisharp and http://www.omnisharp.net. Jonathan Channon has a great overview blog post you should check out that talks about how Jason Imison created OmniSharpServerwhich is an…
HTTP wrapper around NRefactory allowing C# editor plugins to be written in any language. NRefactory is the C# analysis library used in the SharpDevelop and MonoDevelop IDEs. It allows applications to easily analyze both syntax and semantics of C# programs. It is quite similar to Microsoft’s Roslyn project; except that it is not a full compiler – NRefactory only analyzes C# code, it does not generate IL code.
OmniSharp runs as its own process and runs a local Nancy-based web api that your editor of choice talks to. If you have an editor that you like to use, why not get involved and make a plugin? Perhaps for Eclipse?
We now have plugins for these editors:
- Sublime
- Brackets from Adobe
- Atom from GitHub
- Emacs
- Vim
And these work on (so far) all platforms! It’s about choice. We wanted to bring more than autocomplete (which is basically “I think you typed that before”) to your editor, instead we want actual type-smart intellisense, as well as more sophisticated features like refactoring, format document, and lots of other stuff you’d expect only to see in Visual Studio.
We also brought in the Sublime Kulture package which gives Sublime users support for ASP.NET 5 (formerly ASP.NET vNext), so they can launch Kestrel (our libuv based local webserver), run Entity Framework migrations, and other arbitrary commands from within Sublime.
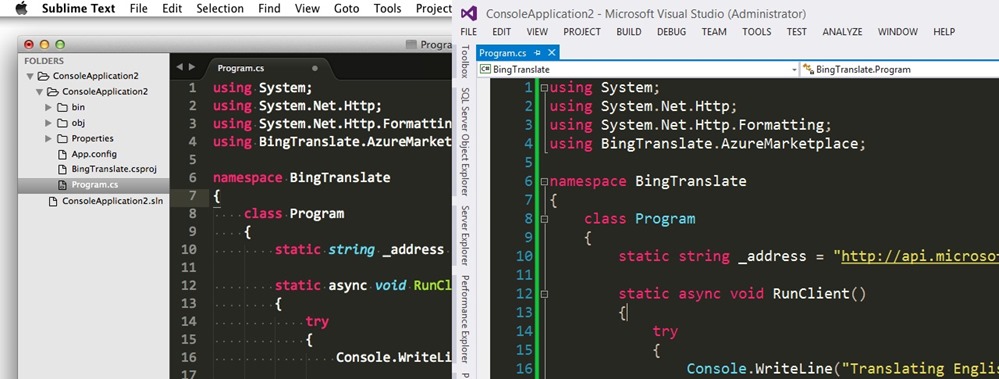
Here’s OmniSharp running in emacs on my Windows machine. The emacs setup (here is an example) is a little more complex than the others, but it also gives emacs folks an extreme level of control. Note that I had to launch the OmniSharp server manually for emacs, while it launches automatically for the other editors.
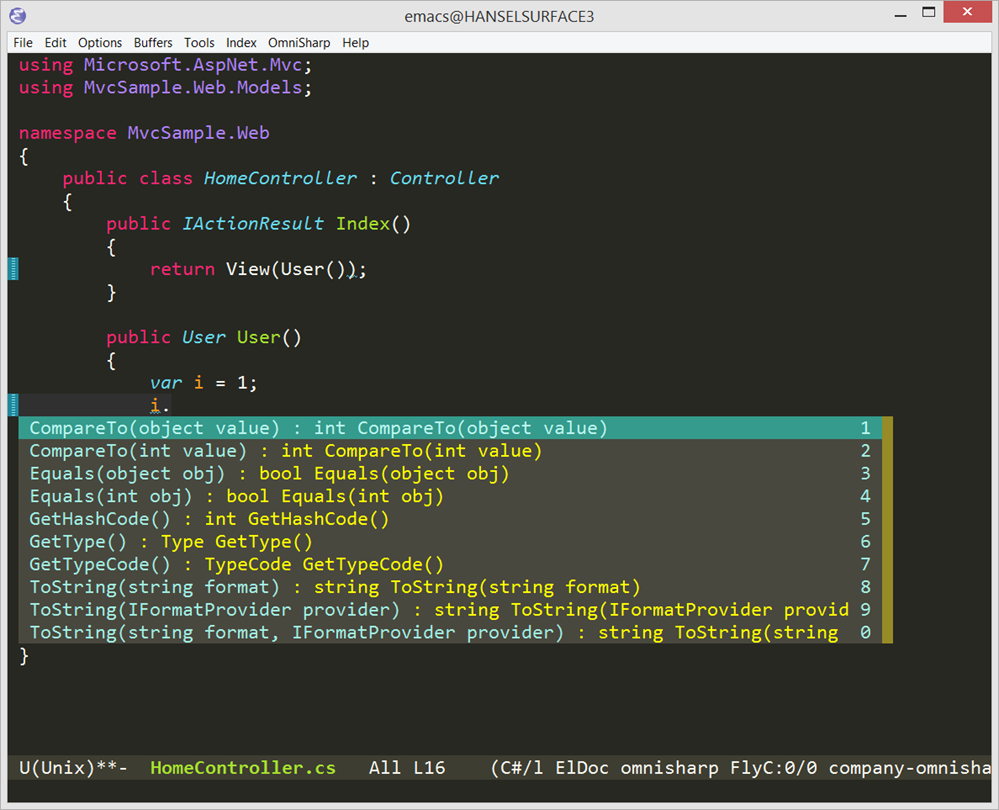
Here is an ASP.NET MVC app running in Sublime. The Sublime OmniSharp package output can be seen in the debug console there (Ctrl+~ to see it).
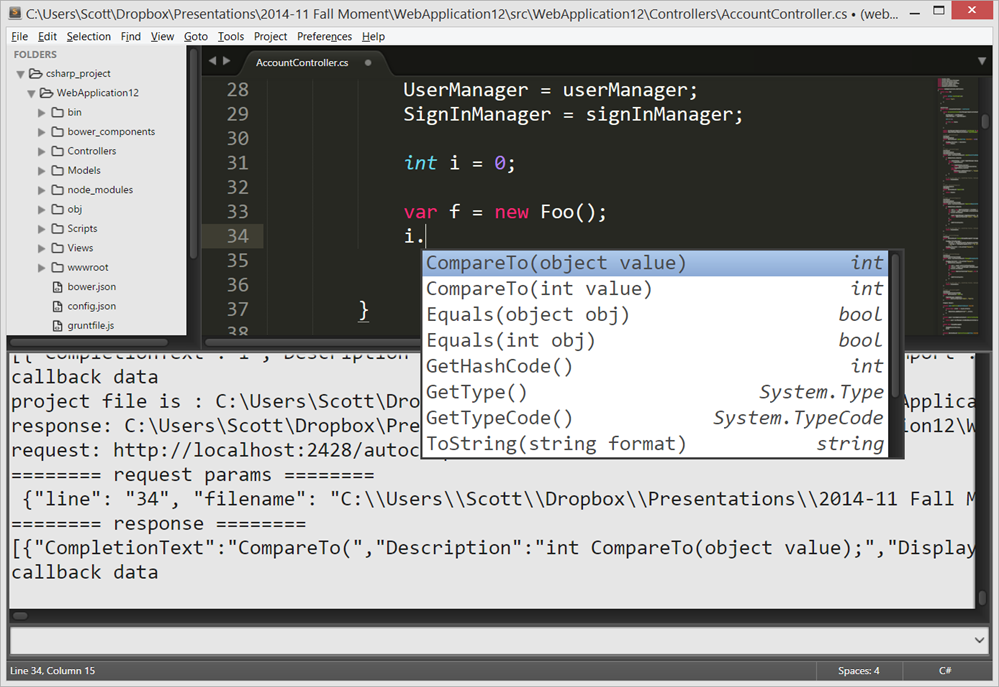
OmniSharp is in very active development. We are looking at bringing in Roslyn, using the new ASP.NET Design Time Host, and improving robustness. It’s not perfect, but it’s pretty darn cool. There’s lots of details in Jonathan’s writeup with great animated gifs showing features. Also note that we have a Yeoman generator for ASP.NET that can get you started when creating ASP.NET 5 apps on Mac or Linux. The yeoman generator can create Console apps, MVC apps, and NancyFx apps.
You can get started at http://omnisharp.net. See you there!
via: http://www.hanselman.com/blog/OmniSharpMakingCrossplatformNETARealityAndAPleasure.aspx